-
-
Save bitinn/72922a0a69f6d723d94e94f2fc21230e to your computer and use it in GitHub Desktop.
A custom node for Unity's ShaderGraph to capture lighting and use it into the shader. Works as of Dec 2018, but the APIs might change!
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using UnityEngine; | |
using UnityEditor.ShaderGraph; | |
using System.Reflection; | |
// IMPORTANT: | |
// - tested with LWRP and Shader Graph 4.6.0-preview ONLY | |
// - likely to break in SG 5.x and beyond | |
// - for HDRP, add your own keyword to detect environment | |
[Title("Custom", "Main Light Data")] | |
public class MainLightDataNode : CodeFunctionNode { | |
// shader code definition | |
// handle shader graph editor environment where main light data isn't defined | |
private static string shaderText = @"{ | |
#ifdef LIGHTWEIGHT_LIGHTING_INCLUDED | |
Light mainLight = GetMainLight(); | |
Color = mainLight.color; | |
Direction = mainLight.direction; | |
Attenuation = mainLight.distanceAttenuation; | |
#else | |
Color = float3(1.0, 1.0, 1.0); | |
Direction = float3(0.0, 1.0, 0.0); | |
Attenuation = 1.0; | |
#endif | |
}"; | |
// disable own preview as no light data in shader graph editor | |
public override bool hasPreview { | |
get { | |
return false; | |
} | |
} | |
// declare node | |
public MainLightDataNode () { | |
name = "Main Light Data"; | |
} | |
// reflection to shader function | |
protected override MethodInfo GetFunctionToConvert () { | |
return GetType().GetMethod( | |
"GetMainLightData", | |
BindingFlags.Static | BindingFlags.NonPublic | |
); | |
} | |
// shader function and port definition | |
private static string GetMainLightData ( | |
[Slot(0, Binding.None)] out Vector3 Color, | |
[Slot(1, Binding.None)] out Vector3 Direction, | |
[Slot(2, Binding.None)] out Vector1 Attenuation | |
) { | |
// define vector3 | |
Direction = Vector3.zero; | |
Color = Vector3.zero; | |
// actual shader code | |
return shaderText; | |
} | |
} |
hi! i am getting 26 errors as well... in 4.6.0 / 4.8.0 / 4.10.0 I tested them all and there is no way to make a build :(
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hi, I'm getting 26 errors keeping me from making a build, on SG 4.10.0 - Perhaps this needs another update?
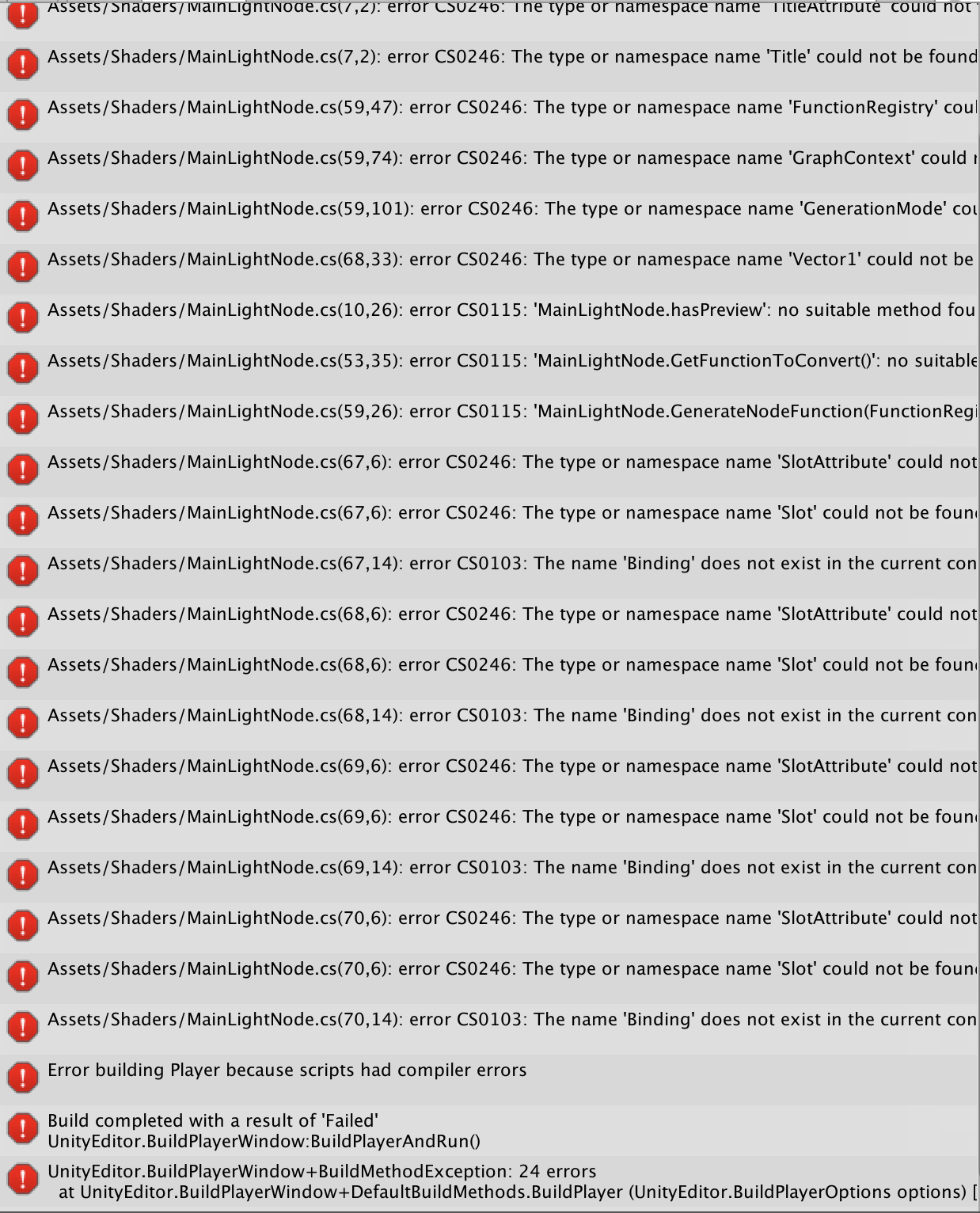