Last active
January 19, 2022 21:27
-
-
Save begin-again/8ffcc6e538b29a2d4c6452ebfdcae30a to your computer and use it in GitHub Desktop.
From a tutorial using electron v9 though trying to get it to work with v12.2.3
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const { app, Menu, Tray } = require('electron'); | |
// eslint-disable-next-line no-unused-vars | |
const MainWindow = require('./MainWindow'); | |
class AppTray extends Tray { | |
/** | |
* Creates an instance of AppTray. | |
* @param {string} icon | |
* @param {MainWindow} mainWindow | |
* @memberof AppTray | |
*/ | |
constructor(icon, mainWindow) { | |
super(icon); | |
this.setToolTip('SysTop'); | |
this.mainWindow = mainWindow; | |
this.on('click', () => { | |
if (this.mainWindow.isVisible() === true) { | |
this.mainWindow.hide(); | |
} else { | |
this.mainWindow.show(); | |
} | |
}); | |
this.on('right-click', () => { | |
const contextMenu = Menu.buildFromTemplate([ | |
{ | |
label: 'Quit', | |
click: () => { | |
app.isQuitting = true; | |
app.quit(); | |
}, | |
}, | |
]); | |
this.popUpContextMenu(contextMenu); | |
}); | |
} | |
} | |
module.exports = AppTray; |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const path = require('path'); | |
const { app, Menu, ipcMain, BrowserWindow } = require('electron'); | |
const Store = require('./Store'); | |
const MainWindow = require('./MainWindow'); | |
const AppTray = require('./AppTray'); | |
// Set env | |
process.env.NODE_ENV = 'development'; | |
const isDev = process.env.NODE_ENV !== 'production' ? true : false; | |
const isMac = process.platform === 'darwin' ? true : false; | |
/** @type {MainWindow} */ | |
let mainWindow; | |
/** @type {AppTray} */ | |
let tray; | |
// Init store & defaults | |
const store = new Store({ | |
configName: 'user-settings', | |
defaults: { | |
settings: { | |
cpuOverload: 80, | |
alertFrequency: 5, | |
}, | |
}, | |
}); | |
function createMainWindow() { | |
mainWindow = new MainWindow('./app/index.html', isDev); | |
} | |
app.on('ready', () => { | |
createMainWindow(); | |
mainWindow.webContents.on('dom-ready', () => { | |
mainWindow.webContents.send('settings:get', store.get('settings')); | |
}); | |
const mainMenu = Menu.buildFromTemplate(menu); | |
Menu.setApplicationMenu(mainMenu); | |
mainWindow.on('close', (e) => { | |
if (!app.isQuitting) { | |
e.preventDefault(); | |
mainWindow.hide(); | |
} | |
return true; | |
}); | |
const icon = path.join(__dirname, 'assets', 'icons', 'tray_icon.png'); | |
// Create tray | |
tray = new AppTray(icon, mainWindow); | |
}); | |
const menu = [ | |
...(isMac ? [{ role: 'appMenu' }] : []), | |
{ | |
role: 'fileMenu', | |
}, | |
{ | |
label: 'View', | |
submenu: [ | |
{ | |
label: 'Toggle Navigation', | |
click: () => mainWindow.webContents.send('nav:toggle'), | |
}, | |
], | |
}, | |
...(isDev | |
? [ | |
{ | |
label: 'Developer', | |
submenu: [ | |
{ role: 'reload' }, | |
{ role: 'forcereload' }, | |
{ type: 'separator' }, | |
{ role: 'toggledevtools' }, | |
], | |
}, | |
] | |
: []), | |
]; | |
// Set settings | |
ipcMain.on('settings:set', (e, value) => { | |
store.set('settings', value); | |
mainWindow.webContents.send('settings:get', store.get('settings')); | |
}); | |
app.on('window-all-closed', () => { | |
if (!isMac) { | |
app.quit(); | |
} | |
}); | |
app.on('activate', () => { | |
if (BrowserWindow.getAllWindows().length === 0) { | |
createMainWindow(); | |
} | |
}); | |
app.allowRendererProcessReuse = true; |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const { BrowserWindow } = require('electron'); | |
class MainWindow extends BrowserWindow { | |
constructor(file, isDev) { | |
super({ | |
title: 'SysTop', | |
width: isDev ? 800 : 355, | |
height: 500, | |
icon: `${__dirname}/assets/icons/icon.png`, | |
resizable: isDev ? true : false, | |
show: false, | |
opacity: 0.9, | |
webPreferences: { | |
nodeIntegration: true, | |
// ideally would like to use contextIsolation but right now this should work | |
contextIsolation: false, | |
}, | |
}); | |
this.loadFile(file); | |
if (isDev) { | |
this.webContents.openDevTools(); | |
} | |
} | |
} | |
module.exports = MainWindow; |
If I move the listeners into the constructor it works,
this.on('click', () => {
if (this.mainWindow.isVisible() === true) {
this.mainWindow.hide();
} else {
this.mainWindow.show();
}
});
this.on('right-click', () => {
const contextMenu = Menu.buildFromTemplate([
{
label: 'Quit',
click: () => {
app.isQuitting = true;
app.quit();
},
},
]);
this.popUpContextMenu(contextMenu);
});
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Getting this error
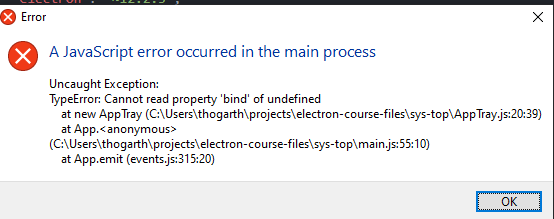