Last active
March 21, 2019 09:34
-
-
Save TenzenIga/0c92066091e4276d40a60f08b9f65737 to your computer and use it in GitHub Desktop.
stringConverter
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* | |
function takes 3 parameters: | |
str - string to convert | |
state - case "up" = uppercase, "low" = lowercase | |
invert(optional) - if true, the first letter will be oposite case | |
*/ | |
function convertString(str, state, invert) { | |
let result = ''; | |
switch (state) { | |
case 'low': | |
for (let i = 0; i < str.length; i++) { | |
result+=toLower(str[i]) | |
} | |
if(invert){ | |
result = toUpper(str[0]) + result.slice(1) | |
} | |
break; | |
case 'up': | |
for (let i = 0; i < str.length; i++) { | |
result +=toUpper(str[i]) | |
} | |
if(invert){ | |
result = toLower(str[0]) + result.slice(1) | |
} | |
break; | |
default: | |
result = 'invalid arguments' | |
break; | |
} | |
return result; | |
} | |
function toUpper(char){ | |
if( char.codePointAt() >= 97 && char.codePointAt() <= 122 ){ // asci range for lowercase characters | |
return String.fromCodePoint(char.codePointAt() - 32) | |
} | |
if( char.codePointAt() >= 1072 && char.codePointAt() <= 1103 ){// acsi range for lower cyrillic characters except 'ё' | |
return String.fromCodePoint(char.codePointAt() - 32) | |
} | |
return char; | |
} | |
function toLower(char){ | |
if( char.codePointAt() >= 65 && char.codePointAt() <= 90 ){ // asci range for uppercase characters | |
return String.fromCodePoint(char.codePointAt()+ 32) | |
} | |
if( char.codePointAt() >= 1040 && char.codePointAt() <= 1071 ){ // acsi range for uppercase cyrillic characters except 'Ё' | |
return String.fromCodePoint(char.codePointAt()+ 32) | |
} | |
return char; | |
} | |
console.log(convertString('Hello', 'up', true)); | |
console.log(convertString('Привет', 'up', true)); | |
console.log(convertString('КАПС ОФФ', 'low')); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Все ок, но Ё не работает))
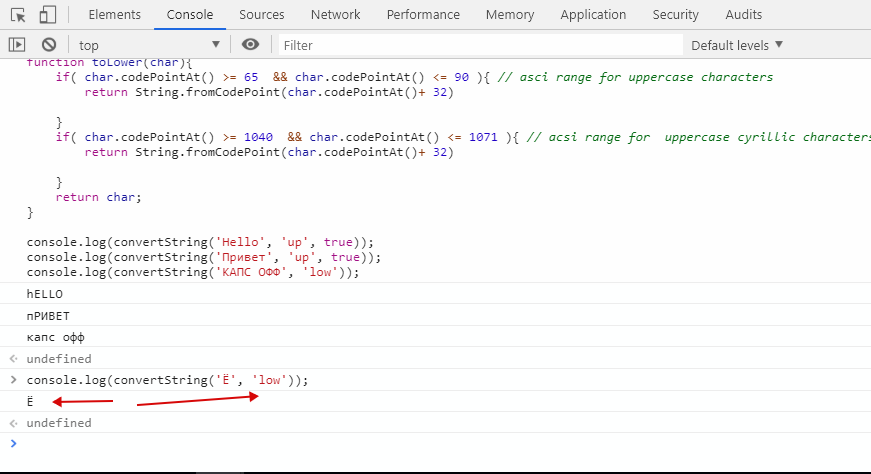