Created
April 26, 2023 10:36
-
-
Save IObert/773709e6058c98a1b63f637a8eac06aa to your computer and use it in GitHub Desktop.
Send Message via Segment Destination Function
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
async function onIdentify(event, settings) { | |
let response; | |
try { | |
const profileApiResponse = await fetch( | |
`https://profiles.segment.com/v1/spaces/${ | |
event.context.personas.space_id | |
}/collections/users/profiles/user_id:${encodeURIComponent( | |
event.userId | |
)}/traits`, | |
{ | |
method: 'GET', | |
headers: { | |
Authorization: `Basic ${btoa(settings.profileApiKey + ':')}` | |
} | |
} | |
); | |
const profile = await profileApiResponse.json(); | |
const traits = profile.traits; | |
response = await fetch( | |
`https://api.twilio.com/2010-04-01/Accounts/${settings.twilioAccountId}/Messages.json`, | |
{ | |
method: 'POST', | |
headers: { | |
Authorization: `Basic ${btoa( | |
settings.twilioAccountId + ':' + settings.twilioAuthToken | |
)}`, | |
'Content-Type': 'application/x-www-form-urlencoded;charset=UTF-8' | |
}, | |
body: toFormParams({ | |
To: traits.isWhatsApp ? `whatsapp:${event.userId}` : event.userId, | |
From: settings.messagingServiceSid, | |
Body: settings.message | |
}) | |
} | |
); | |
} catch (error) { | |
// Retry on connection error | |
throw new RetryError(error.message); | |
} | |
if (response.status >= 500 || response.status === 429) { | |
// Retry on 5xx (server errors) and 429s (rate limits) | |
throw new RetryError(`Failed with ${response.status}`); | |
} | |
} | |
function toFormParams(params) { | |
return Object.entries(params) | |
.map(([key, value]) => { | |
const paramName = encodeURIComponent(key); | |
const param = encodeURIComponent(value); | |
return `${paramName}=${param}`; | |
}) | |
.join('&'); | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
This function first retrieves the traits from the Profile API and then uses the Twilio API to send either a SMS or a WhatsApp message:
This is the needed config:
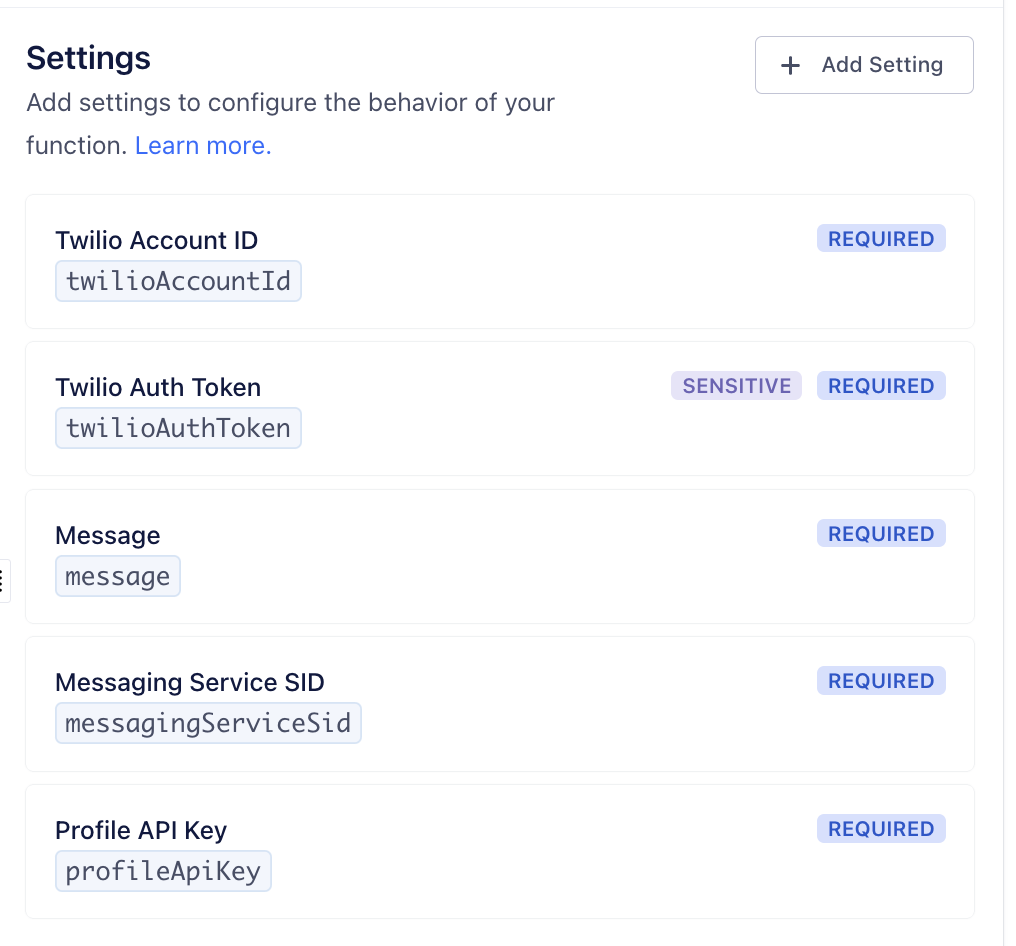